Arduino/Projects/ClassBuzzers
WIP: This project has not been finished, or hardly started. This is a dump of researched information.
The 433MHz receivers purchased for the arduino are able to receive codes sent from 433MHz remotes such as these.
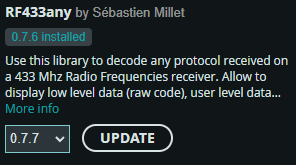
The library RF433any is highly recommended for any project using these receivers (or transmitters). See screenshot on the right.
The library contains several very useful examples. You can use the example 03_output-signal-timings.ino to get the codes used by the remote, and reference 05_callback.ino for how to create callbacks for different codes. Make sure you use the correct encoding; the example uses RF433ANY_ID_TRIBIT_INV but in my experience the remotes tend to use RF433ANY_ID_TRIBIT instead. Example 03 will tell you which one. Note that the examples use the uncommon serial baud 115200.
Receiving codes
My recommendation is to do something like the following (not tested but should at least be very close):
#include "RF433any.h" #include <Arduino.h> #define PIN_RFINPUT 2 Track track(PIN_RFINPUT); BitVector* allCodes[][4] = { // Note that 0x4f == 0b01001111 so the first bit does not count. There are only 23 bits here, not 24. // 0xff and 0xf2 on the other hand begin the byte with a 1 not a 0 and so they will be a full 24 bits. // This is only relevant for the first byte. { new BitVector(24, 3, 0xff, 0x7f, 0x23), new BitVector(24, 3, 0xf2, 0x7f, 0x23), new BitVector(23, 3, 0x4f, 0x7f, 0x23), new BitVector(23, 3, 0x4f, 0x7f, 0x28) }, { new BitVector(24, 3, 0xf9, 0x7f, 0x23), new BitVector(24, 3, 0xf2, 0x3f, 0x23), new BitVector(23, 3, 0x4f, 0x7e, 0x23), new BitVector(23, 3, 0x4f, 0x7f, 0xcc) }, }; byte dummy; void on_call_anycode(void *data) { } void on_call(void *data) { byte n = (byte *)data - &dummy; Serial.print("Received code number "); Serial.println(n); int playerNumber = n / 4 + 1; int playerChoice = n % 4; Serial.print("Player buzz: "); Serial.println(playerNumber); Serial.print("Player choice: "); Serial.println((char) (((byte) "A") + playerChoice)); } void setup() { pinMode(PIN_RFINPUT, INPUT); Serial.begin(115200); track.setopt_wait_free_433_before_calling_callbacks(true); for (int i = 0; i < sizeof(allCodes) / sizeof(BitVector) / 4; i++) { for (int w = 0; w < 4; w++) { track.register_callback(RF433ANY_ID_TRIBIT, allCodes[i][w], (void *)(&dummy + 4*i+w), on_call, 100); } } } void loop() { track.treset(); while (!track.do_events()) delay(1); }
Programming codes to the remote
Very little work has been done here. You'll need to set the remote to programming mode, then transmit the desired code from the arduino transmitter module at the same time as pressing the desired button on the remote. You can't use the RF433any library for that, you'll need to use a different one. Make sure you choose a library that allows you to transmit raw binary data and not encoded messages as the encoded messages contain metadata that will throw off what you think you're sending.